When an HTML document's DOM structure is retrieved using JavaScript, the structure often contains empty nodes.
For example, given a document like this:
<html>
<body>
<div>
<h1>foo</h1>
<div>
<ul>
<li>bar</li>
</ul>
</div>
</div>
</body>
</html>
Squish will get a DOM structure similar to this one from the browser:
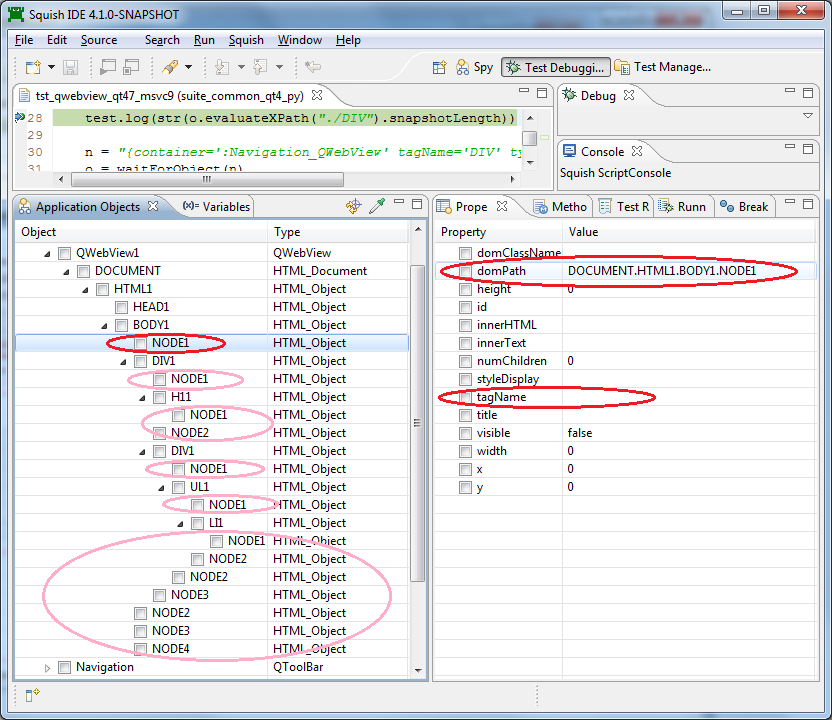
The empty nodes are called NODE1
, NODE2
, etc.
Here is a little example that shows how to retrieve the children of a specific object and how to remove the empty nodes from that list:
def main():
startBrowser("https://www.froglogic.com")
o = waitForObject({"id": "squishplatforms", "tagName": "UL"})
children = object.children(o)
test.log("Child count with NODEs: " + str(len(children)))
children2 = removeNODEs(children)
test.log("Child count without NODEs: " + str(len(children2)))
def removeNODEs(objs):
res = []
for o in objs:
if hasattr(o, "tagName") and o.tagName != "undefined":
res.append(o)
return res
Expected output:
Child count with NODEs: 17
Child count without NODEs: 8